Python for Beginners: Python has solidified itself as one of the most popular programming languages globally. This versatile language, known for its readability and beginner-friendly syntax, empowers individuals of all backgrounds to enter the exciting world of coding. Whether you’re a student, aspiring developer, or simply curious about programming, Python offers a smooth entry point for your coding journey.
This comprehensive guide serves as your stepping stone to mastering the fundamentals of Python programming. We’ll delve into the core concepts, explore practical coding exercises, and equip you with the knowledge to confidently navigate your initial Python projects.
Python for Beginners
Why Python? Understanding the Appeal
Before we dive into the specifics, let’s explore what makes Python such a compelling choice for beginners:
Readability:
Python code resembles plain English, making it easier to understand and write compared to languages with complex syntax.
Versatility:
Python’s extensive libraries and frameworks empower you to tackle various tasks, from web development and data analysis to machine learning and scientific computing.
Large and Supportive Community:
Python boasts a vast and active community of developers, offering unparalleled support and resources for beginners.
Free and Open-Source:
Python is completely free to use and modify, eliminating financial barriers to entry.
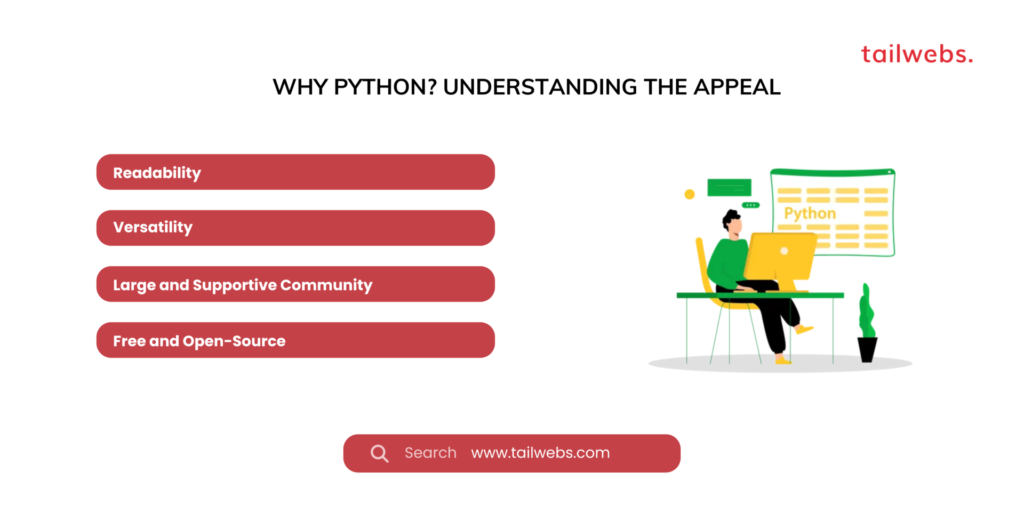
Setting Up Your Python Development Environment
The first step towards your Python coding adventure is establishing your development environment. Here’s a breakdown of the essential tools:
Python Interpreter:
Download and install the latest version of the Python interpreter from https://www.python.org/downloads/. This software executes your Python code.
Code Editor or IDE (Integrated Development Environment):
While you can write Python code in a simple text editor, using a code editor or IDE offers enhanced features like syntax highlighting, code completion, and debugging tools. Popular options include Visual Studio Code, PyCharm, and Thonny (ideal for beginners).
Let’s Get Coding: Your First Python Program
It’s time to write your first Python program! Here’s a classic example to get you started:
print("Hello, World!")
Save this code as a .py
file (e.g., hello_world.py) and run it using your Python interpreter. You should see the message “Hello, World!” printed on your screen. Congratulations, you’ve just written and executed your first Python program!
Python for beginners:
Understanding Core Python Concepts
Now that you’ve experienced the thrill of coding, let’s delve into some fundamental Python concepts:
Variables and Data Types:
Variables store data within your program, and data types define the kind of data a variable can hold. Python offers basic data types like integers (whole numbers), floats (decimal numbers), strings (text), and booleans (True or False).
Example of variables and data types
name = “Alice” # String
age = 30 # Integer
pi = 3.14159 # Float
is_coding = True # Boolean
Operators:
Operators perform operations on data. Python provides arithmetic operators (+, -, *, /), comparison operators (==, !=, <, >, <=, >=), and logical operators (and, or, not).
Example of operators
result = 10 + 5 # Arithmetic operator (addition)
is_equal = name == “Alice” # Comparison operator (equal to)
is_adult = age >= 18 # Logical operator (greater than or equal to)
Input and Output:
Your program can interact with the user by accepting input and displaying output. Python provides functions like input()
to get user input and print()
to display messages.
Example of input and output
user_name = input(“Enter your name: “)
print(“Hello,”, user_name)
Control Flow Statements:
Control flow statements dictate how your program executes by allowing you to control the sequence of code execution. Conditional statements (if, else, elif) enable you to make decisions based on certain conditions, while loops (while, for) allow for repeated execution of code blocks.
Example of control flow statements (if statement)
age = int(input(“Enter your age: “))
if age >= 18:
print(“You are an adult.”)
else:
print(“You are not an adult.”)
Practice Makes Perfect: Essential Beginner Exercises
The best way to solidify your understanding is through practice. Here are some practical exercises to help you master these core concepts:
Number Guessing Game:
Let the computer generate a random number between 1 and 100, and allow the user to guess the number within a limited number of attempts. Provide feedback to the user based on their guess (too high, too low, correct).
Mad Libs Generator:
Create a program that prompts the user for various words (e.g., noun, verb, adjective) and then inserts them into a pre-written story template, resulting in a funny or nonsensical story.
Simple Calculator:
Build a basic calculator that allows users to perform addition, subtraction, multiplication, and division on two user-provided numbers.
Text Analyzer:
Write a program that accepts a string of text from the user and performs basic analysis. Count the number of words, characters, vowels, and consonants in the text.
These exercises allow you to experiment with the core concepts you’ve learned and gain hands-on experience with writing Python code.
Introducing Functions: Building Reusable Code Blocks
Functions are reusable blocks of code that perform a specific task. They promote code modularity, improve readability, and prevent code duplication. Here’s how to define and use functions in Python:
def greet(name):
“””This function greets the user by name.”””
print(“Hello,”, name)
Calling the function
greet(“Bob”)
In this example, the greet
function takes a name as input and prints a personalized greeting message. You can call this function multiple times with different names to reuse the same functionality.
Practice Makes Perfect: Exercises with Functions
Area Calculator:
Create a function that calculates the area of a rectangle or circle based on user-provided dimensions (length and breadth for rectangle, radius for circle). Call this function from your main program to calculate the area for different shapes.
Temperature Converter:
Write a function that converts Celsius to Fahrenheit or vice versa based on user input. Utilize this function within your program to offer temperature conversion functionality.
By incorporating functions into your Python programs, you’ll structure your code more effectively and enhance its reusability.
Essential Resources to Fuel Your Python Learning
Official Python Tutorial:
The official Python tutorial from docs.python.org serves as a comprehensive resource for beginners, covering the fundamentals in detail with clear explanations and code examples. https://docs.python.org/3/tutorial/
Learn Python the Hard Way:
This online book by Zed Shaw offers a more hands-on approach, emphasizing practice and problem-solving through a series of exercises. https://learnpythonthehardway.org/
Codecademy’s Learn Python 3 Course:
Codecademy provides an interactive platform to learn Python through a series of bite-sized coding exercises and challenges. https://www.codecademy.com/learn/learn-python-3
Coursera’s Python for Everybody Specialization:
Coursera offers a well-structured online specialization course from the University of Michigan, covering Python programming fundamentals and data structures. https://www.coursera.org/specializations/python
These resources cater to different learning styles and preferences, allowing you to choose the ones that best suit your needs.
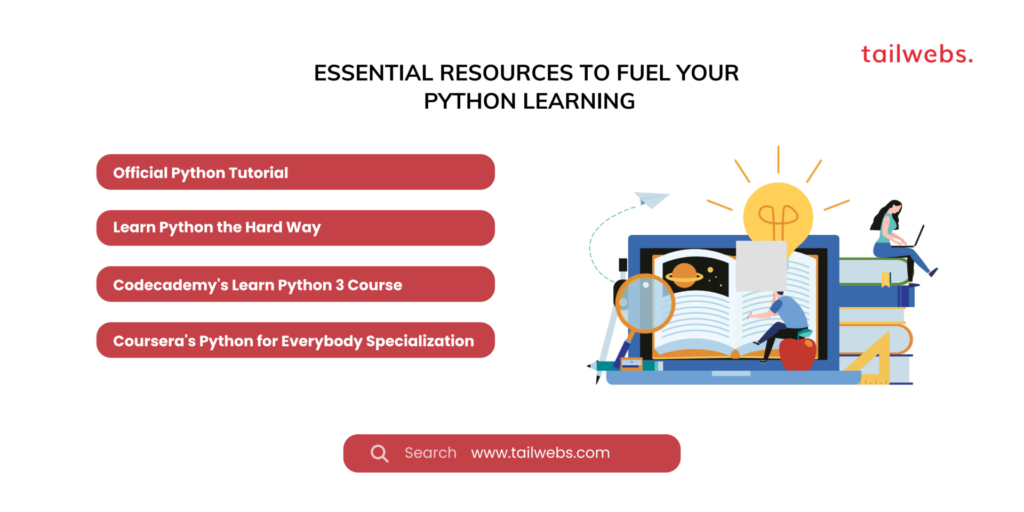
The Power of Community: Connect with Fellow Python Learners
The Python community is vast and supportive. Here are some ways to connect with other learners and get help:
Online Forums:
Stack Overflow is a popular forum where you can post your Python coding questions and receive solutions and guidance from experienced developers. https://stackoverflow.com/
Reddit Communities:
Subreddits like r/learnpython offer a platform to connect with fellow beginners, share learning experiences, and ask questions.
Local Meetups:
Many cities host Python meetups where you can network with other Python enthusiasts, attend workshops, and learn from each other.
Data Structures: The Building Blocks of Organized Data
Data structures are fundamental components in programming that organize and store data in a specific way. Python provides various built-in data structures to efficiently manage different types of data:
Lists:
Ordered, mutable collections of items enclosed in square brackets []
. Lists can hold elements of different data types.
fruits = [“apple”, “banana”, “cherry”]
numbers = [1, 2, 3, 4, 5]
mixed_list = [10.5, “hello”, True]
Tuples:
Ordered, immutable collections of items enclosed in parentheses ()
. Once created, the elements within a tuple cannot be changed.
fruits_tuple = (“apple”, “banana”, “cherry”)
coordinates = (3, 5)
Dictionaries:
Unordered collections of key-value pairs enclosed in curly braces {}
. Dictionaries provide a flexible way to associate unique keys with their corresponding values.
person = {“name”: “Alice”, “age”: 30, “city”: “New York”}
Sets:
Unordered collections of unique elements enclosed in curly braces {}
. Sets eliminate duplicate values.
letters = {“a”, “b”, “c”, “b”} # Duplicate “b” will be removed
Understanding these data structures will empower you to manage and manipulate data effectively within your Python programs.
Object-Oriented Programming (OOP): Organizing Code with Classes and Objects
Object-oriented programming (OOP) is a programming paradigm that revolves around objects, which encapsulate data (attributes) and related operations (methods). This approach promotes code reusability, modularity, and maintainability.
Classes:
A blueprint or template that defines the properties (attributes) and functionalities (methods) of objects.
class Car:
def init(self, brand, model, color): # Constructor method
self.brand = brand
self.model = model
self.color = color
def accelerate(self):
print(“The car is accelerating!”)
def brake(self):
print(“The car is braking!”)
my_car = Car(“Ford”, “Mustang”, “Red”)
my_car.accelerate() # Calling a method on the object
Objects:
Instances created from a class definition. Each object has its own set of attributes and can access the methods defined within the class.
By leveraging OOP principles, you can structure your Python programs for more efficient code organization and reusability.
Modules and Packages: Sharing and Reusing Code
As your projects grow, managing code efficiently becomes crucial. Python offers modules and packages to organize and share code:
Modules:
Python files containing reusable functions, variables, and classes. Modules can be imported into other Python programs to utilize their functionalities.
Create a module named math_functions.py
def add(x, y):
return x + y
def subtract(x, y):
return x – y
Packages:
Create a directory named calculations
Inside calculations, create init.py (an empty file)
Move math_functions.py to the calculations directory
Import the module from the package
from calculations import add, subtract
result = add(10, 5)
print(result)
Beyond the Basics: Exploring Advanced Python Concepts
Once you’ve grasped these intermediate topics, you can delve into more advanced areas of Python programming:
- Exception Handling: Techniques for managing errors and preventing program crashes.
- File Handling: Reading from and writing data to files.
- Regular Expressions: Powerful tools for pattern matching and text manipulation.
- Web Scraping: Extracting data from websites programmatically.
- Databases and SQL: Interacting with databases using Python.
- GUI Development: Building graphical user interfaces (GUIs) for your applications.
- Machine Learning and Data Science: Utilizing Python for data analysis, machine learning algorithms, and data visualization.
The Gratification of Continuous Learning: Your Python Journey Awaits
By diligently working through the concepts and exercises presented in this guide, you’ve established a solid foundation in Python programming. Remember, the key to mastering any skill is consistent practice and exploration. Here are some tips to fuel your ongoing Python learning journey:
Start Small and Build Gradually:
Don’t be intimidated by complex projects. Begin with smaller, manageable programs and progressively increase the difficulty as your skills develop.
Embrace Challenges:
Step outside your comfort zone and tackle problems that require you to learn new concepts. This is how you’ll expand your knowledge and problem-solving abilities.
Personalize Your Learning:
Explore areas of Python that pique your interest. Whether it’s web development, data analysis, or machine learning, delve into topics that motivate you.
Contribute to Open-Source Projects:
Consider contributing to open-source Python projects on platforms like GitHub. This allows you to collaborate with other developers, gain practical experience, and build your portfolio.
Stay Updated:
The world of technology is constantly evolving. Stay informed about the latest Python trends, libraries, and frameworks by following blogs, attending conferences, and participating in online communities.
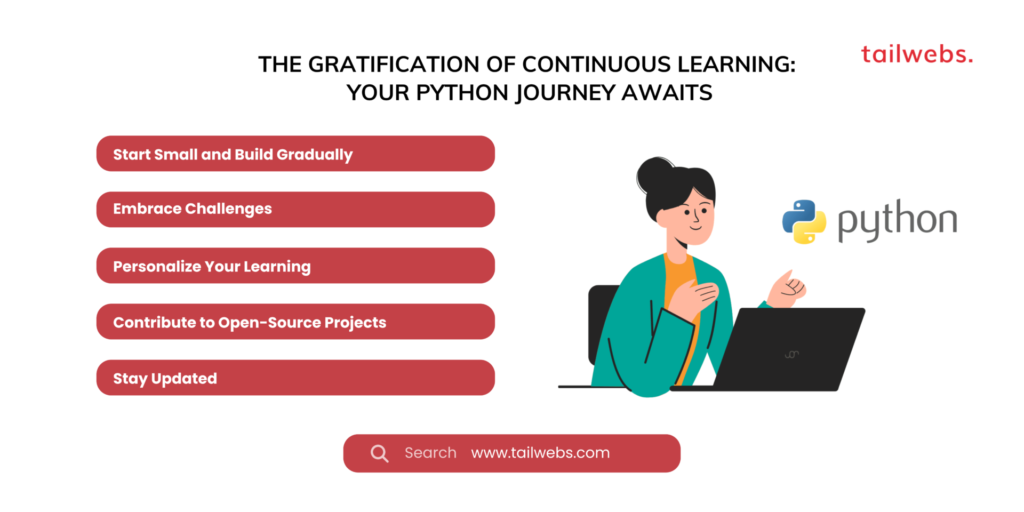
Conclusion: Python, Your Gateway to a Fulfilling Programming Career
Python has opened its doors to the exciting world of programming. As you continue your Python endeavors, you’ll unlock a vast array of possibilities. From crafting web applications and automating tasks to exploring data science and machine learning, Python empowers you to turn your ideas into reality.
This guide serves as a stepping stone on your Python programming journey. Embrace the challenges, celebrate your achievements, and never stop learning. With dedication and perseverance, you’ll transform your Python proficiency into a valuable asset, propelling you towards a fulfilling career in the ever-growing tech landscape.